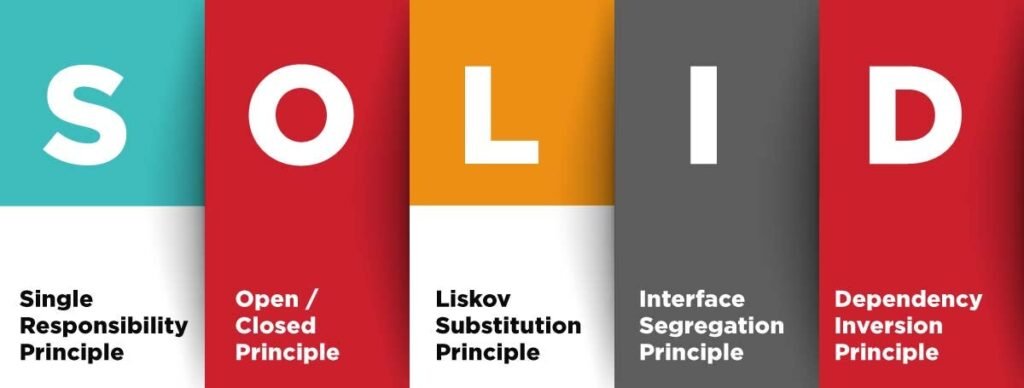
The Single Responsibility Principle (SRP) is a design principle in software engineering that states that a class or module should have only one reason to change. In other words, a class or module should have a single responsibility or job, and it should encapsulate that responsibility completely.
The SRP is one of the five principles of SOLID, a set of design principles that aim to make software systems more maintainable, flexible, and understandable. By adhering to the SRP, you can achieve better code organization, reusability, and testability.
The key idea behind the SRP is that a class or module should have a clear and well-defined responsibility, which usually corresponds to a specific functionality or concern within the system. By separating responsibilities, you can isolate changes related to a specific aspect of the system, reducing the impact on other parts.
Benefits of applying the SRP include
- Increased maintainability: When a class has only one responsibility, it becomes easier to understand, modify, and debug. Changes related to that responsibility are less likely to affect other parts of the system, reducing the risk of unintended consequences.
- Improved reusability: A class that is focused on a single responsibility can be more easily reused in different contexts or projects. It becomes a building block that can be combined with other components to create more complex systems.
- Enhanced testability: Classes with a single responsibility are typically easier to test because their behavior is focused and well-defined. Unit testing becomes simpler, as you can isolate the class’s responsibility and test it independently.
However, it’s important to note that the SRP does not mean that a class or module should have only one method or be limited to a certain number of lines of code. It’s about ensuring that the responsibilities of a class are cohesive and aligned with the Single Responsibility Principle.
The SRP states that a class or module should have only one reason to change. In other words, it should have a single responsibility or job. The responsibility refers to a specific aspect of the system’s functionality, and the class or module should encapsulate that responsibility completely.
Key Points
Here are some key points to understand about the Single Responsibility Principle
- Separation of concerns: The SRP aims to separate different concerns or functionalities into separate classes or modules. By doing so, each class can focus on its specific responsibility, making the codebase easier to understand and maintain.
- High cohesion: Cohesion refers to how closely the members of a class or module are related to each other. The SRP promotes high cohesion by ensuring that a class has a well-defined, singular responsibility. High cohesion leads to more modular and understandable code.
- Low coupling: Coupling refers to the degree of dependency between different classes or modules. By adhering to the SRP, you reduce the coupling between classes since each class has a clear boundary and limited responsibilities. Low coupling increases the flexibility and reusability of code.
- Adapting to change: When a class or module has a single responsibility, changes related to that responsibility can be localized, making the system more adaptable. Modifying one responsibility should not affect other unrelated parts of the system, minimizing the risk of unintended side effects.
- Granularity and balance: Applying the SRP requires finding the right balance in class design. It’s important to choose a level of granularity that is appropriate for the complexity of the system. Too fine-grained classes may lead to excessive proliferation, while classes with too many responsibilities become harder to maintain.
- Collaborative relationships: Classes that follow the SRP tend to have well-defined interfaces and can collaborate effectively with other classes. The separation of concerns allows for better encapsulation, modular design, and the ability to compose different components to build complex systems.
It’s worth noting that the SRP applies not only to classes but also to modules, functions, and other components of a software system. It encourages breaking down complex systems into smaller, manageable parts that have clear responsibilities.
Restriction of single responsibility principle
While the Single Responsibility Principle (SRP) is a valuable design principle, it does have certain limitations and potential pitfalls that developers should be aware of. Here are some restrictions or challenges associated with the SRP:
- Subjective definition of responsibility: Determining what constitutes a single responsibility for a class or module can sometimes be subjective. Different developers may have varying interpretations of what constitutes a single responsibility, leading to differing designs. It requires careful analysis and judgment to identify the appropriate responsibilities for a class.
- Balancing granularity: Achieving the right level of granularity for classes can be challenging. If classes become too fine-grained and each handles a very small responsibility, it can lead to an excessive number of classes, which can be cumbersome to manage and understand. On the other hand, having classes with too many responsibilities violates the SRP and can result in less maintainable code.
- Dependencies between responsibilities: In some cases, different responsibilities may have dependencies on each other, making it difficult to separate them into distinct classes without introducing complex relationships or excessive coupling. Care must be taken to strike a balance between maintaining the SRP and ensuring practicality in the design.
- Duplication of code: Following the SRP may result in a certain amount of code duplication, as responsibilities are separated into different classes. Duplication can be mitigated through appropriate use of techniques like inheritance, composition, or the extraction of shared components. However, it requires careful consideration to avoid excessive duplication and maintain code coherence.
- Overemphasis on SRP at the expense of other principles: While the SRP is important, focusing solely on it without considering other design principles and trade-offs can lead to rigid and overly complex designs. It is essential to strike a balance with other principles such as cohesion, coupling, and code reuse to ensure a well-rounded and maintainable system.
- Limited scope of SRP: The SRP primarily focuses on individual classes or modules. It does not address larger-scale architectural concerns or interactions between components. Applying additional architectural patterns and design principles can help address these broader concerns.
Despite these limitations, the SRP remains a valuable principle for designing maintainable and flexible software systems. It provides a guideline for structuring classes and modules around specific responsibilities, promoting code organization and reducing the risk of unintended consequences when making changes. However, it should be applied judiciously, considering the specific context and requirements of the software project.
What Business Problem Solves SRP
The Single Responsibility Principle (SRP) is primarily a design principle in software engineering, rather than a direct solution to a specific business problem. However, adhering to the SRP can help address several business-related challenges and improve the overall effectiveness of software development. Here are some business problems that the SRP can help mitigate:
- Maintainability and scalability: As software systems grow in size and complexity, maintaining and scaling them becomes increasingly challenging. By following the SRP, classes or modules have well-defined responsibilities, making it easier to understand, modify, and extend the system. This leads to improved maintainability, reducing the time and effort required for ongoing development and enhancements.
- Code reusability: Reusability is a crucial aspect of software development as it helps save time and effort by leveraging existing code components. When classes adhere to the SRP, they become more modular and focused, making them easier to reuse in different contexts. This promotes code reusability across projects, reducing development costs and improving productivity.
- Testing and debugging: The SRP promotes better testability by ensuring that each class has a clear and well-defined responsibility. Classes with single responsibilities are easier to test in isolation, enabling more focused unit testing. This simplifies the debugging process as issues can be localized to specific responsibilities, making it easier to identify and fix problems.
- Agility and adaptability: In today’s fast-paced business environment, the ability to quickly adapt and respond to changes is critical. When classes have single responsibilities, modifications related to a specific responsibility have minimal impact on other parts of the system. This promotes agility, allowing businesses to implement changes faster and respond to evolving market needs more effectively.
- Collaboration and development efficiency: By following the SRP, classes or modules become more self-contained and focused. This improves collaboration among development teams as different team members can work on different responsibilities independently. It also enables parallel development and reduces the likelihood of conflicts and merge issues, enhancing overall development efficiency.
- System stability and fault isolation: The SRP helps minimize the risk of unintended consequences when making changes to the system. By separating responsibilities, the impact of modifications is limited to a specific area, reducing the likelihood of introducing bugs or causing system-wide failures. This improves system stability and facilitates fault isolation, making it easier to identify and address issues.
Pros of the Single Responsibility Principle:
- Enhanced maintainability: By adhering to the SRP, classes or modules have a clear and well-defined responsibility. This makes the codebase easier to understand, modify, and maintain. Changes related to a specific responsibility have minimal impact on other parts of the system, reducing the risk of unintended consequences and making maintenance more efficient.
- Improved reusability: Classes that follow the SRP are often more modular and focused. This promotes code reusability as individual classes can be easily extracted and reused in different contexts or projects. Reusing well-defined responsibilities can save development time, reduce code duplication, and improve overall productivity.
- Better testability: When a class has a single responsibility, it becomes easier to write focused and targeted unit tests. Testing a class in isolation is simpler and more effective, as there are fewer interactions and dependencies to consider. This leads to more comprehensive test coverage, better bug detection, and improved software quality.
- Reduced coupling: The SRP encourages low coupling between classes. Each class focuses on its specific responsibility and has minimal dependencies on other classes. This loose coupling enhances code flexibility, as changes to one responsibility are less likely to ripple through the entire system. It promotes better modularity and makes the codebase more flexible and adaptable to change.
- Clearer code organization: The SRP helps in organizing the codebase by grouping related responsibilities together. This provides a clear structure and separation of concerns, making the codebase more understandable and easier to navigate. It enhances the overall readability of the code and facilitates collaboration among team members.
Cons of the Single Responsibility Principle:
- Increased number of classes: Applying the SRP can result in a larger number of classes or modules, especially in complex systems. Each responsibility is encapsulated in a separate class, which can lead to a proliferation of classes. This can make the codebase more complex and harder to manage, especially if the granularity of responsibilities is not balanced properly.
- Potential code duplication: Separating responsibilities into different classes may lead to duplication of code, especially if there are shared functionalities between responsibilities. Managing and synchronizing similar code across multiple classes can be challenging and may introduce maintenance overhead.
- Subjective determination of responsibilities: Identifying and defining the appropriate responsibilities for each class can be subjective and open to interpretation. Different developers may have different views on what constitutes a single responsibility, which can lead to inconsistencies in the design. It requires careful analysis and collaboration to establish a shared understanding of responsibilities.
- Balancing granularity: Striking the right balance in the granularity of responsibilities can be challenging. Fine-grained responsibilities can result in an excessive number of small classes, potentially increasing complexity and making the system harder to understand. On the other hand, coarse-grained responsibilities can violate the SRP and make classes harder to maintain and modify.
- Potential overemphasis on SRP: Focusing solely on the SRP without considering other design principles and trade-offs can lead to rigid designs or an excessive decomposition of responsibilities. It’s important to consider other principles like cohesion, coupling, and code reuse to achieve a well-rounded and maintainable system.
Overall, while the Single Responsibility Principle offers several benefits, it’s important to carefully consider the specific context and trade-offs involved. The application of the SRP should be balanced with other design principles and the needs of the software system.
Example : SWIFT
// Example of a class violating the SRP
class Customer {
var name: String
var email: String
init(name: String, email: String) {
self.name = name
self.email = email
}
func save() {
// Code to save customer data to a database
// This responsibility is unrelated to the customer's data representation
}
func sendEmail(message: String) {
// Code to send an email to the customer
// This responsibility is unrelated to the customer's data representation
}
}
In the above code, the Customer
class violates the SRP because it has multiple responsibilities. It not only represents customer data but also includes methods to save the customer data to a database and send emails to the customer. Let’s refactor this code to adhere to the SRP:
// Refactored code following the SRP
struct Customer {
var name: String
var email: String
}
class CustomerRepository {
func save(customer: Customer) {
// Code to save customer data to a database
}
}
class EmailService {
func sendEmail(to email: String, message: String) {
// Code to send an email to the given email address
}
}
Example : Objective-C
// Example of a class violating the SRP
@interface Customer : NSObject
@property (nonatomic, strong) NSString *name;
@property (nonatomic, strong) NSString *email;
- (void)save;
- (void)sendEmailWithMessage:(NSString *)message;
@end
@implementation Customer
- (void)save {
// Code to save customer data to a database
// This responsibility is unrelated to the customer's data representation
}
- (void)sendEmailWithMessage:(NSString *)message {
// Code to send an email to the customer
// This responsibility is unrelated to the customer's data representation
}
@end
In the above code, the Customer
class violates the SRP because it has multiple responsibilities. It not only represents customer data but also includes methods to save the customer data to a database and send emails to the customer. Let’s refactor this code to adhere to the SRP:
// Refactored code following the SRP
@interface Customer : NSObject
@property (nonatomic, strong) NSString *name;
@property (nonatomic, strong) NSString *email;
@end
@interface CustomerRepository : NSObject
- (void)saveCustomer:(Customer *)customer;
@end
@implementation CustomerRepository
- (void)saveCustomer:(Customer *)customer {
// Code to save customer data to a database
}
@end
@interface EmailService : NSObject
- (void)sendEmailTo:(NSString *)email withMessage:(NSString *)message;
@end
@implementation EmailService
- (void)sendEmailTo:(NSString *)email withMessage:(NSString *)message {
// Code to send an email to the given email address
}
@end
In the refactored code, we have separated the responsibilities into distinct classes. The Customer
class now solely represents the customer data. The CustomerRepository
class is responsible for saving customer data to a database, and the EmailService
class handles sending emails.
Conclusion
In conclusion, the Single Responsibility Principle (SRP) is a fundamental design principle in software engineering that promotes the concept of “one class, one responsibility.” It suggests that a class or module should have a single, well-defined responsibility, which helps improve the maintainability, reusability, and testability of the codebase.
Overall, the SRP is a valuable principle for designing maintainable and modular software systems. By separating concerns and ensuring each class has a single responsibility, developers can create codebases that are easier to understand, maintain, and extend. It promotes code organization, collaboration, and long-term system stability, contributing to the overall success of software projects.
Happy Coding 🙂