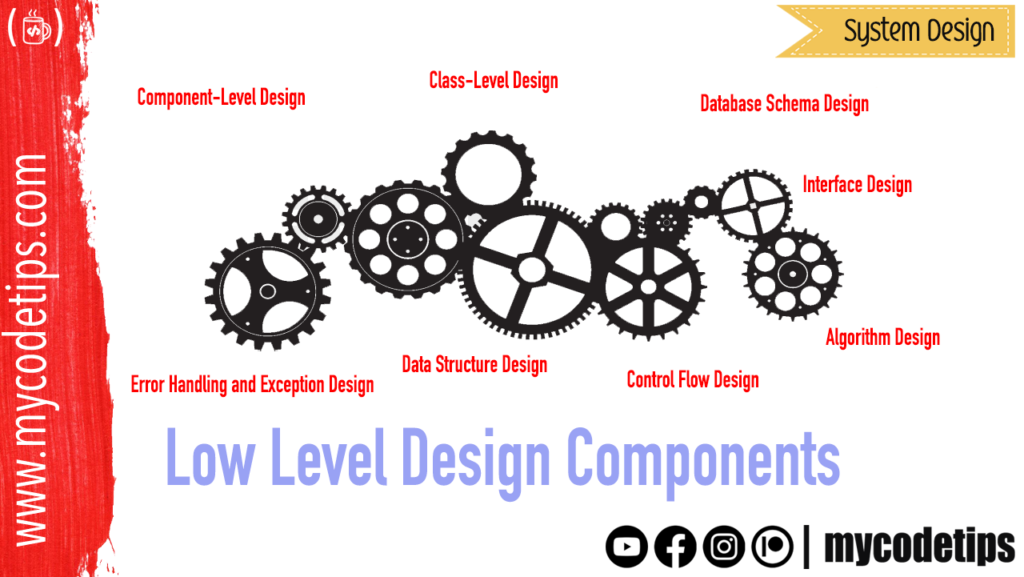
Business Problems Solves in Low Level Design : Low-level design (LLD) is a phase in software development where the high-level architectural design is further refined into detailed specifications and implementation plans. It focuses on the internal components, modules, classes, and algorithms required to implement the system or software application. LLD provides a blueprint for developers to write code and guides the implementation phase.
During the low-level design phase, the system’s functionality is divided into smaller units, and each unit is designed in detail. The design decisions made in this phase directly impact the code structure, algorithms, data structures, and interfaces used in the implementation.
Key activities in the low-level design phase include:
- Detailed Component Design: Each component or module identified in the high-level design is further decomposed into smaller units. Detailed designs are created for each unit, specifying the internal structure, relationships, data flows, and interfaces.
- Algorithm and Data Structure Design: Detailed algorithms are designed to implement specific functionalities. Data structures are selected and defined based on the system’s requirements and performance considerations.
- Interface Design: The interfaces between different components or modules are defined, including the methods, parameters, and data types exchanged. This includes specifying input/output formats, error handling mechanisms, and communication protocols.
- Database Schema Design: If the system involves a database, the database schema is designed, including tables, fields, relationships, and constraints.
- Control Flow Design: Detailed control flow diagrams, such as sequence diagrams or flowcharts, are created to illustrate the step-by-step execution of the system’s processes and functions.
- Error Handling and Exception Design: Strategies for error handling, exception management, and recovery mechanisms are defined to ensure system stability and resilience.
- Performance and Optimization Considerations: Design decisions are made to optimize performance, minimize resource usage, and address scalability and efficiency requirements.
- Security and Validation Design: Security mechanisms, data validation techniques, and access control measures are designed to ensure the system’s security and integrity.
Different Types of LLD
There are different types or levels of Low-Level Design (LLD) based on the scope and granularity of the design details. The specific types may vary depending on the software development methodology and the complexity of the system. Here are a few common types of LLD:
- Component-Level Design: This type of LLD focuses on the detailed design of individual components or modules within the system. It includes defining the internal structure, data structures, algorithms, interfaces, and relationships of each component.
- Class-Level Design: Class-level design is concerned with the detailed design of classes and their relationships within a component or module. It involves specifying the attributes, methods, and properties of each class, along with their access levels and dependencies on other classes.
- Data Structure Design: In systems that heavily rely on data processing or storage, data structure design is an important aspect of LLD. It involves designing the data structures, such as arrays, lists, queues, trees, or graphs, based on the requirements of the system and the efficient manipulation and storage of data.
- Database Schema Design: LLD may include the design of the database schema if the system involves data storage. This includes defining tables, relationships, keys, indexes, constraints, and data types in a relational database or determining the structure and organization of data in a NoSQL database.
- Interface Design: LLD includes the design of interfaces between components, modules, or external systems. This includes specifying the methods, parameters, return types, and communication protocols used for interaction.
- Algorithm Design: LLD may involve the design of algorithms for specific functionalities or operations within the system. This includes selecting appropriate algorithms, defining the step-by-step procedures, considering performance and efficiency factors, and addressing edge cases.
- Control Flow Design: LLD encompasses the design of control flow within the system, depicting the sequence of operations, branching, loops, and decision-making processes. This can be represented using flowcharts, sequence diagrams, or state diagrams.
- Error Handling and Exception Design: LLD includes the design of error handling mechanisms and exception management. This involves identifying potential error scenarios, defining error codes or types, specifying error messages, and outlining the steps for error detection, reporting, and recovery.
Here’s an example of Low-Level Design (LLD) for a simple banking system. Please note that this is a simplified example to illustrate the concept and may not cover all aspects of a real-world banking system:
- Component-Level Design: Components:
- User Interface
- Account Management
- Transaction Processing
- Authentication Detailed design for each component includes specifying internal structures, data flows, and interfaces.
- Class-Level Design: Classes for the “Account Management” component:
- Account: Represents a bank account with properties like account number, balance, and account holder details. It includes methods for deposit, withdrawal, and balance inquiry.
- Customer: Represents a customer with properties like name, address, and contact information. It includes methods for managing customer details.
- AccountRepository: Handles the storage and retrieval of account information from a database or file system.
- CustomerRepository: Handles the storage and retrieval of customer information.
- AccountService: Implements business logic for account-related operations. Similar class-level designs are created for other components.
- Data Structure Design: Data structures for the “Transaction Processing” component:
- Transaction: Represents a single transaction with properties like transaction ID, account number, transaction type, amount, and timestamp.
- Database Schema Design: Database schema for the banking system:
- Account table: Contains columns like account number, balance, account holder details.
- Customer table: Contains columns like customer ID, name, address, and contact information.
- Transaction table: Contains columns like transaction ID, account number, transaction type, amount, and timestamp.
- Interface Design: Interfaces between components:
- AccountManagementUI: Defines methods for displaying account-related information and capturing user input.
- AccountServiceInterface: Defines methods for account-related operations like deposit, withdrawal, and balance inquiry.
- Algorithm Design: Algorithm for transaction processing:
- PerformTransaction: Implements the logic for processing different transaction types (deposit, withdrawal, etc.), updating the account balance, and recording the transaction details.
- Control Flow Design: Control flow diagrams or sequence diagrams are created to illustrate the step-by-step execution of various processes within the system, such as account creation, transaction processing, or customer information update.
- Error Handling and Exception Design: Design strategies for handling errors and exceptions, such as validating user input, handling insufficient balance, or addressing database connectivity issues.
Advantages of LLD
Low-Level Design (LLD) offers several benefits in software development. Here are some of the key advantages of LLD:
- Improved Code Quality: LLD helps ensure that the codebase is well-structured, modular, and follows design principles. By defining detailed specifications and implementation plans, LLD encourages clean code, proper organization of classes and modules, and adherence to coding standards.
- Enhanced Maintainability: With a well-defined LLD, it becomes easier to understand and modify the system. Changes or enhancements can be made more efficiently because the design decisions and dependencies are documented. LLD promotes modularity, which enables developers to isolate and update specific components without affecting the entire system.
- Scalability and Extensibility: LLD allows for better scalability and extensibility of the software. By designing components and modules with clear interfaces and separation of concerns, adding new features or integrating additional functionality becomes more manageable. LLD helps identify potential points of extension, making the system more flexible and adaptable to future requirements.
- Better Collaboration: LLD serves as a blueprint that facilitates collaboration among developers, architects, and stakeholders. It provides a common understanding of the system’s design, behavior, and requirements. With a clear LLD, team members can work together more effectively, discuss design decisions, and ensure consistency across the implementation.
- Testability: LLD promotes testability by allowing for easier isolation and unit testing of individual components. Well-defined interfaces and clear dependencies make it simpler to create test cases and mock dependencies. By designing for testability during LLD, developers can identify potential areas of complexity or coupling that might hinder testing.
- Performance Optimization: LLD provides an opportunity to consider performance optimizations early in the development process. By carefully designing algorithms, data structures, and control flows, developers can analyze potential performance bottlenecks and make informed design decisions to improve system performance.
- Reduced Development Time: While LLD adds an extra step in the development process, it can ultimately save time in the long run. By having a well-thought-out design and clear specifications, developers can proceed with implementation more efficiently, avoiding costly rework or refactoring caused by unclear requirements or ad-hoc design decisions.
- Systematic Troubleshooting: When issues or bugs arise, LLD can serve as a reference point for troubleshooting. By understanding the system’s design and dependencies, developers can trace problems to their source more effectively, identify the impacted components, and implement fixes in a targeted manner.
Drawbacks or Disadvantage of LLD
While Low-Level Design (LLD) offers numerous benefits, there are also some potential drawbacks and challenges that can arise. Here are a few cons of LLD:
- Increased Development Time: LLD involves a detailed analysis and design phase, which adds additional time to the overall development process. It requires careful consideration of design decisions, documentation, and coordination among team members. This can lead to a longer development cycle, especially for complex systems or projects with tight deadlines.
- Over-Engineering: Excessive focus on LLD can sometimes result in over-engineering. Developers may spend excessive time designing intricate architectures, creating complex class hierarchies, or over-optimizing code, which can lead to unnecessary complexity and reduced productivity. It’s important to strike a balance between detailed design and practicality.
- Difficulty in Handling Changes: While LLD promotes maintainability, it can still pose challenges when it comes to accommodating changes or evolving requirements. If the design is too rigid or tightly coupled, making modifications to the system may require extensive refactoring or impact multiple components. Adapting the LLD to accommodate changes may involve additional effort and can introduce the risk of introducing new bugs.
- Design and Implementation Mismatch: There is always a risk of a mismatch between the LLD and the actual implementation. The design may be based on assumptions or incomplete information, and during implementation, developers may discover limitations or unforeseen complexities. This may require revisiting and updating the LLD, leading to additional effort and potential inconsistencies.
- Potential Overhead: Depending on the size and complexity of the project, maintaining detailed design documentation for LLD can introduce administrative overhead. Keeping the design artifacts up-to-date, managing changes, and ensuring that the documentation remains relevant can be challenging and time-consuming.
- Limited Flexibility: A highly detailed LLD can sometimes limit flexibility and innovation during the implementation phase. Developers may feel restricted by the predefined design decisions and find it difficult to introduce alternative approaches or technologies that may better address the requirements. This can hinder creativity and experimentation.
- Learning Curve and Collaboration Challenges: LLD documentation can be complex and require a certain level of expertise to understand and interpret. New team members joining the project may need time to grasp the design concepts and the relationships between components. Additionally, collaborating on a highly detailed LLD may require additional effort to ensure that everyone is on the same page and working towards a shared understanding.
Key points of Low-Level Design (LLD) include:
- Detailed Specifications: LLD involves specifying detailed specifications and implementation plans for the system’s components, modules, classes, algorithms, and interfaces. It provides a blueprint for developers to follow during the implementation phase.
- Component Decomposition: LLD breaks down the system’s functionality into smaller units, such as components or modules. Each unit is designed in detail, specifying its internal structure, relationships, data flows, and interfaces.
- Class and Interface Design: LLD focuses on designing classes and their relationships within components. It includes defining attributes, methods, properties, access levels, and dependencies. Interface design involves specifying the methods, parameters, return types, and communication protocols exchanged between components.
- Data Structure Design: LLD may involve designing data structures based on the system’s requirements and efficient data manipulation and storage. This includes selecting appropriate data structures such as arrays, lists, queues, trees, or graphs.
- Algorithm Design: LLD includes designing algorithms to implement specific functionalities or operations within the system. It involves selecting suitable algorithms, defining step-by-step procedures, and considering performance and efficiency factors.
- Control Flow Design: LLD defines the control flow within the system, depicting the sequence of operations, branching, loops, and decision-making processes. This can be represented using flowcharts, sequence diagrams, or state diagrams.
- Error Handling and Exception Design: LLD addresses strategies for error handling, exception management, and recovery mechanisms. It includes identifying potential error scenarios, defining error codes or types, specifying error messages, and outlining steps for error detection, reporting, and recovery.
- Database Schema Design: If the system involves data storage, LLD may include designing the database schema. This includes defining tables, fields, relationships, keys, indexes, and constraints.
- Performance Considerations: LLD provides an opportunity to consider performance optimizations early in the development process. It involves designing algorithms, data structures, and control flows to enhance system performance, minimize resource usage, and address scalability requirements.
- Collaboration and Documentation: LLD serves as a reference for collaboration among developers, architects, and stakeholders. It facilitates a common understanding of the system’s design and requirements. LLD documentation includes detailed design documents, class diagrams, sequence diagrams, data flow diagrams, and database schemas.
Some commonly used graphical representations for Low-Level Design (LLD) that you can create to visually represent the design:
- Class Diagrams: Class diagrams illustrate the classes, their attributes, methods, and relationships within the system. They use boxes to represent classes and arrows to depict relationships such as inheritance, association, or dependency.
- Sequence Diagrams: Sequence diagrams show the interaction between different components or objects in a sequential manner. They depict the flow of messages exchanged between objects over time, highlighting the order of method invocations and responses.
- Data Flow Diagrams: Data flow diagrams (DFDs) visualize the flow of data within the system. They show how data is input, processed, and output by various components, modules, or functions. DFDs use circles or rectangles to represent processes and arrows to represent the data flow.
- State Diagrams: State diagrams illustrate the different states and transitions of an object or system. They show how the system or object moves from one state to another based on certain events or conditions.
- Entity-Relationship Diagrams: Entity-relationship diagrams (ERDs) represent the database schema and relationships between entities. They depict tables, attributes, primary keys, foreign keys, and the associations between different tables.
- Flowcharts: Flowcharts represent the flow of control or processes within the system. They use various shapes such as rectangles for processes, diamonds for decision points, and arrows to depict the flow of control or data.
- Structure Charts: Structure charts depict the structure of a system or program, showing the hierarchy of modules or components. They use boxes to represent modules or components and lines to represent the relationships or dependencies between them.
Business Problems Solves in Low Level Design
The business problem that LLD helps solve is ensuring the successful and efficient development of complex software or systems. Here are some specific business problems that LLD can address:
- System Architecture: LLD allows businesses to define the overall structure and organization of their software or system. It helps determine how different components will interact and communicate with each other, ensuring that the system meets the desired functionality and performance requirements.
- Component Integration: LLD ensures that individual components can seamlessly integrate and work together within the larger system. It defines the interfaces, protocols, and data formats used for communication between components, enabling smooth interoperability.
- Performance Optimization: LLD enables businesses to identify potential performance bottlenecks and optimize the system’s design accordingly. It allows for fine-tuning of algorithms, data structures, and resource allocation to ensure efficient processing and minimize response times.
- Scalability and Extensibility: LLD considers future growth and requirements by designing the system in a scalable and extensible manner. It enables businesses to add new features or functionality to the system without significant rework or disruption.
- Maintainability and Modularity: LLD promotes a modular design approach, making it easier to maintain and enhance the system over time. It allows for encapsulation of functionality within individual components, facilitating code reuse, troubleshooting, and overall system stability.
- Risk Mitigation: LLD helps identify potential risks and challenges associated with the system’s implementation. By carefully designing and planning the low-level details, businesses can minimize the likelihood of errors, security vulnerabilities, and system failures.
In conclusion, Low-Level Design (LLD) is a critical phase in the software or system development process that focuses on the detailed design and specification of individual components or modules. LLD addresses the technical aspects of system development and helps solve various business problems. It ensures the successful implementation of complex software or systems by addressing system architecture, component integration, performance optimization, scalability, extensibility, maintainability, modularity, and risk mitigation.
Happy Coding 🙂