- The Android build system compiles app resources and source code, and packages them into APKs that you can test, deploy, sign, and distribute.
- Android Studio uses Gradle, an advanced build toolkit, to automate and manage the build process, while allowing you to define flexible custom build configurations.
- Each build configuration can define its own set of code and resources, while reusing the parts common to all versions of your app.
- The Android plugin for Gradle works with the build toolkit to provide processes and configurable settings that are specific to building and testing Android applications.
build process
The build process involves many tools and processes that convert your project into an Android Application Package (APK).
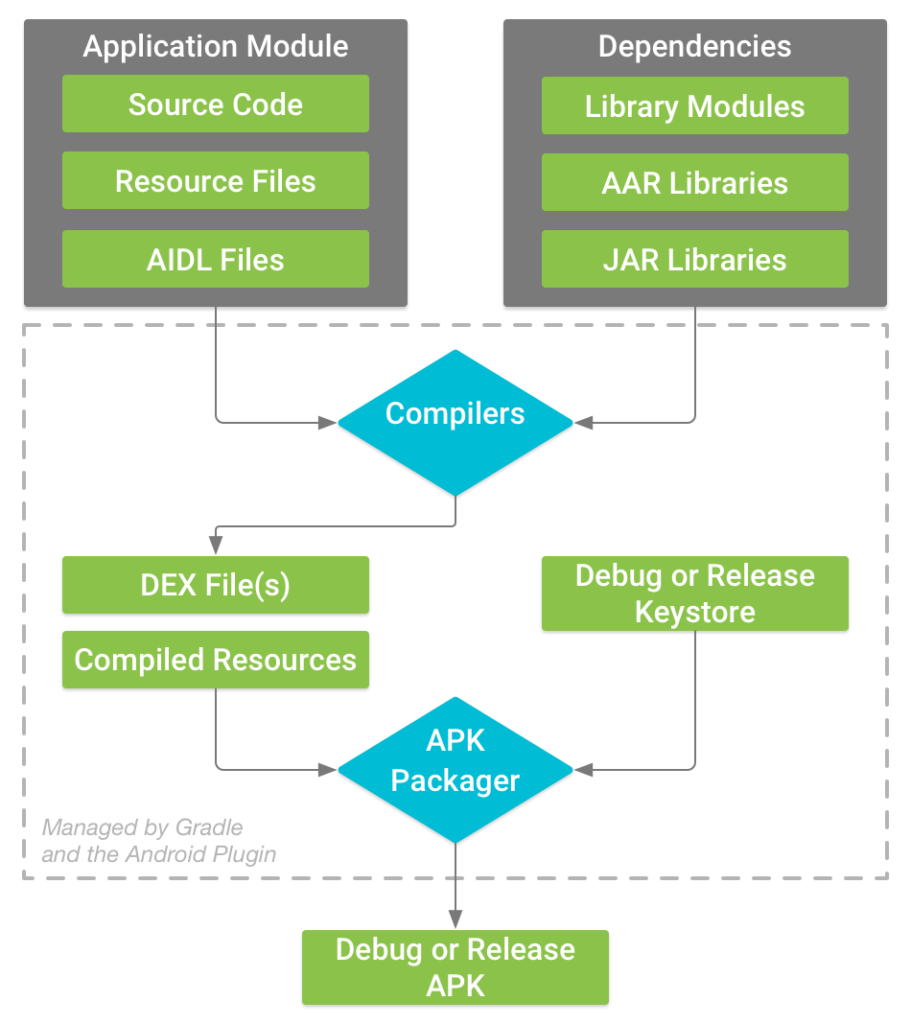
- The compilers convert your source code into DEX (Dalvik Executable) files, which include the bytecode that runs on Android devices, and everything else into compiled resources.
- The APK Packager combines the DEX files and compiled resources into a single APK. Before your app can be installed and deployed onto an Android device, however, the APK must be signed.
- The APK Packager signs your APK using either the debug or release keystore:
- If you are building a debug version of your app, that is, an app you intend only for testing and profiling, the packager signs your app with the debug keystore. Android Studio automatically configures new projects with a debug keystore.
- If you are building a release version of your app that you intend to release externally, the packager signs your app with the release keystore. To create a release keystore, read about signing your app in Android Studio.
- Before generating your final APK, the packager uses the zipalign tool to optimize your app to use less memory when running on a device.
Gradle and the Android plugin
Gradle and the Android plugin help you configure the following aspects of your build:
Build types
Build types define certain properties that Gradle uses when building and packaging your app, and are typically configured for different stages of your development lifecycle
Product flavors
Product flavors represent different versions of your app that you may release to users, such as free and paid versions of your app.
Build variants
A build variant is a cross product of a build type and product flavor, and is the configuration Gradle uses to build your app.
Manifest entries
You can specify values for some properties of the manifest file in the build variant configuration. These build values override the existing values in the manifest file.
Dependencies
The build system manages project dependencies from your local filesystem and from remote repositories.
Signing
The build system enables you to specify signing settings in the build configuration, and it can automatically sign your APKs during the build process.
Code and resource shrinking
The build system enables you to specify a different ProGuard rules file for each build variant.
Multiple APK support
The build system enables you to automatically build different APKs that each contain only the code and resources needed for a specific screen density or Application Binary Interface (ABI).
Build configuration files
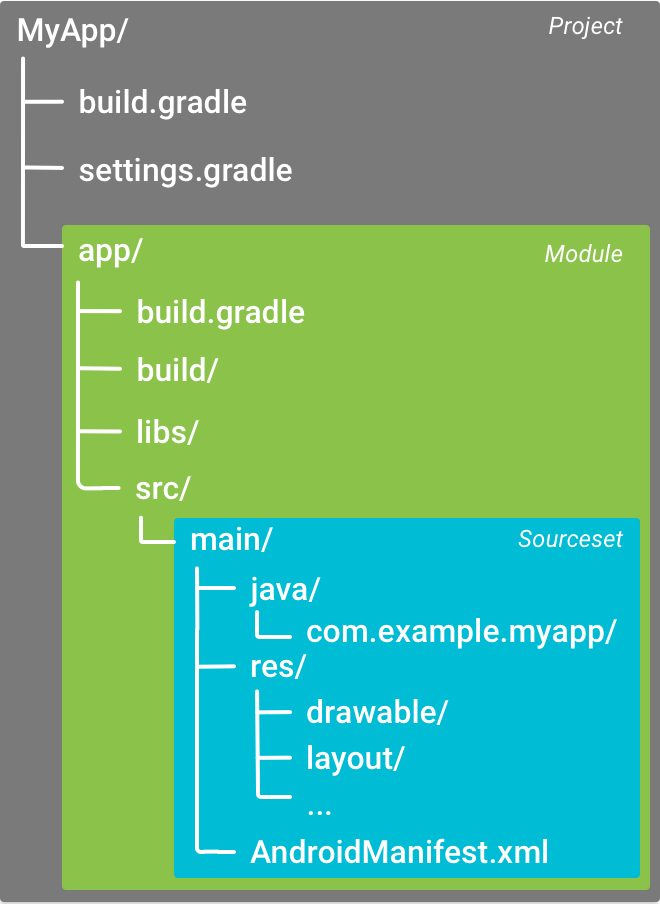
Creating custom build configurations requires you to make changes to one or more build configuration files, or build.gradle files.
When starting a new project, Android Studio automatically creates some of these files for you
Gradle settings file
The settings.gradle file, located in the root project directory, tells Gradle which modules it should include when building your app.
[Groovy]
include ‘:app’
[kotlin]
include(":app")
Top-level build file
The top-level build.gradle file, located in the root project directory, defines build configurations that apply to all modules in your project. By default, the top-level build file uses the buildscript block to define the Gradle repositories and dependencies that are common to all modules in the project.
[Groovy]
buildscript { repositories { google() jcenter() } dependencies { classpath 'com.android.tools.build:gradle:4.2.0' } } allprojects { repositories { google() jcenter() } }
[kotlin]
buildscript { repositories { google() jcenter() } dependencies { classpath("com.android.tools.build:gradle:4.2.0") } } allprojects { repositories { google() jcenter() } }
Configure project-wide properties
For Android projects that include multiple modules, it may be useful to define certain properties at the project level and share them across all the modules.
[Groovy]
buildscript {...} allprojects {...} ext { sdkVersion = 28 supportLibVersion = "28.0.0" ... } ...
[kotlin]
buildscript {...} allprojects {...} extra["compileSdkVersion"] = 28 extra["supportLibVersion"] = "28.0.0" ... } ...
The module-level build file
The module-level build.gradle file, located in each project/module/ directory, allows you to configure build settings for the specific module it is located in.
[Groovy]
apply plugin: 'com.android.application' android { compileSdkVersion 28 buildToolsVersion "30.0.2" defaultConfig { applicationId 'com.example.myapp' // Defines the minimum API level required to run the app. minSdkVersion 15 // Specifies the API level used to test the app. targetSdkVersion 28 // Defines the version number of your app. versionCode 1 // Defines a user-friendly version name for your app. versionName "1.0" } buildTypes { release { minifyEnabled true // Enables code shrinking for the release build type. proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } flavorDimensions "tier" productFlavors { free { dimension "tier" applicationId 'com.example.myapp.free' } paid { dimension "tier" applicationId 'com.example.myapp.paid' } } } dependencies { implementation project(":lib") implementation 'com.android.support:appcompat-v7:28.0.0' implementation fileTree(dir: 'libs', include: ['*.jar']) }
[kotlin]
plugins { id("com.android.application") } android { compileSdkVersion(28) buildToolsVersion = "30.0.2" defaultConfig { applicationId = "com.example.myapp" // Defines the minimum API level required to run the app. minSdkVersion(15) // Specifies the API level used to test the app. targetSdkVersion(28) // Defines the version number of your app. versionCode = 1 // Defines a user-friendly version name for your app. versionName "1.0" } buildTypes { getByName("release") { minifyEnabled = true // Enables code shrinking for the release build type. proguardFiles( getDefaultProguardFile("proguard-android.txt"), "proguard-rules.pro" ) } } flavorDimensions = "tier" productFlavors { create("free") { dimension = "tier" applicationId = "com.example.myapp.free" } create("paid") { dimension = "tier" applicationId = "com.example.myapp.paid" } } } dependencies { implementation(project(":lib")) implementation("com.android.support:appcompat-v7:28.0.0") implementation(fileTree(mapOf("dir" to "libs", "include" to listOf("*.jar")))) }
Gradle properties files
Gradle also includes two properties files, located in your root project directory, that you can use to specify settings for the Gradle build toolkit itself:
gradle.properties
local.properties
Configures local environment properties for the build system, including the following:
- ndk.dir – Path to the NDK. This property has been deprecated. Any downloaded versions of the NDK will be installed in the ndk directory within the Android SDK directory.
- sdk.dir – Path to the SDK.
- cmake.dir – Path to CMake.
- ndk.symlinkdir – in Android Studio 3.5+, creates a symlink to the NDK that can be shorter than the installed NDK path.
Syncing project with Gradle files
When you make changes to the build configuration files in your project, Android Studio requires that you sync your project files.
To sync your project files, click Sync Now in the notification bar that appears when you make a change
Source sets
Android Studio logically groups source code and resources for each module into source sets.
src/main/
This source set includes code and resources common to all build variants.
src/buildType/
Create this source set to include code and resources only for a specific build type.
src/productFlavor/
Create this source set to include code and resources only for a specific product flavor.
src/productFlavorBuildType/
Create this source set to include code and resources only for a specific build variant.
build variant > build type > product flavor > main source set > library dependencies
The moral of the Story of Gradle is.
This allows Gradle to use files that are specific to the build variant you are trying to build while reusing activities, application logic, and resources that are common to other versions of your app.
Happy Coding 🙂